OAuth vulnerability in Chrome extensions: Sounds boring, right? Wrong. Think of it as a backdoor to your digital life, quietly letting malicious actors snoop on your data. These seemingly harmless browser add-ons, often boasting convenient features, can harbor serious security flaws, leveraging the OAuth 2.0 protocol to gain unauthorized access to your sensitive information – from your emails to your social media accounts. We’re diving deep into the murky depths of these vulnerabilities, exploring how they work, how to spot them, and most importantly, how to protect yourself.
Chrome extensions, those handy little tools that streamline our browsing experience, often rely on OAuth 2.0 for authentication. This protocol allows extensions to access user data from various services without directly handling passwords. However, poorly implemented OAuth within these extensions creates significant security risks. Malicious actors can exploit vulnerabilities to steal sensitive data, impersonate users, or even take complete control of accounts. This article unpacks the technical intricacies, exposes common vulnerabilities, and provides actionable steps to secure your Chrome extension ecosystem.
OAuth 2.0 in Chrome Extensions: Oauth Vulnerability In Chrome Extensions
Chrome extensions often need to access data from external websites on behalf of the user. This is where OAuth 2.0 comes in, providing a secure and standardized way to grant limited access without sharing the user’s full credentials. Understanding how OAuth 2.0 functions within the Chrome extension ecosystem is crucial for both developers and users alike.
OAuth 2.0 allows Chrome extensions to access user data from various services without directly handling sensitive information like passwords. Instead, it uses access tokens, which are temporary credentials granted by the service provider after successful authentication. This protects user privacy and simplifies the development process for extensions.
OAuth 2.0 Flow in Chrome Extensions
The OAuth 2.0 flow in a Chrome extension typically involves several steps. First, the extension redirects the user to the authorization server of the service they want to access (e.g., Google Drive, Gmail). The user logs in and grants the extension specific permissions. Upon successful authentication, the authorization server redirects the user back to the extension with an authorization code. The extension then exchanges this code for an access token, using its client ID and secret (which should be kept securely). This access token is used to make subsequent API calls to the service on behalf of the user. The entire process is designed to be transparent to the user, yet secure.
Permissions Requested by Extensions
Chrome extensions requesting access via OAuth 2.0 must clearly specify the permissions needed. These permissions directly impact user privacy and security. For instance, an email extension might request permission to “read email,” while a calendar extension might ask for “read and write calendar events.” Overly broad permissions should raise red flags, as they could potentially expose more user data than necessary. Users should carefully review the requested permissions before granting access to any extension. Granting excessive permissions might allow the extension to access more information than intended, leading to privacy concerns.
Step-by-Step Guide: OAuth 2.0 Usage in Chrome Extensions
A typical implementation involves these steps:
- Registration: The extension developer registers their application with the service provider (e.g., Google, Facebook) to obtain a client ID and client secret.
- Authorization Request: The extension redirects the user to the service provider’s authorization endpoint with parameters including the client ID, redirect URI, and requested scopes (permissions).
- User Authentication: The user logs in to the service provider and grants the extension the requested permissions.
- Authorization Code: The service provider redirects the user back to the extension’s redirect URI with an authorization code.
- Token Exchange: The extension uses the authorization code, client ID, and client secret to request an access token from the service provider’s token endpoint.
- API Access: The extension uses the access token to make API calls to the service provider’s API to access user data.
- Token Refresh: Access tokens typically expire. The extension can use a refresh token (obtained during the token exchange) to obtain a new access token without requiring the user to re-authenticate.
Comparison of OAuth 2.0 Grant Types
Different grant types offer varying levels of security and user experience. The choice depends on the specific needs of the extension.
Grant Type | Description | Security | User Experience |
---|---|---|---|
Authorization Code | Most secure; uses a secure server-side exchange for the access token. | High | Requires a redirect, slightly more complex. |
Implicit | Simpler; returns the access token directly in the redirect. | Lower (access token exposed in URL) | Simpler, faster. |
Client Credentials | Used for machine-to-machine communication; no user interaction. | Moderate | No user interaction needed. |
Resource Owner Password Credentials | Least secure; extension directly receives the user’s password. Generally discouraged. | Low | Simplest, but highly insecure. |
Common Vulnreabilities in OAuth Implementation within Chrome Extensions
Chrome extensions, while offering enhanced browsing experiences, often interact with external services using OAuth 2.0 for authentication. However, improper implementation of OAuth within these extensions can create significant security vulnerabilities, exposing user data and potentially leading to devastating consequences. Let’s dive into some of the most common pitfalls.
Lack of Proper Authorization and Scope Management
Poorly defined authorization scopes are a major vulnerability. If an extension requests overly broad permissions during the OAuth flow, it gains access to more user data than strictly necessary. This excess access can be exploited by malicious actors to steal sensitive information, even if the extension itself isn’t inherently malicious. A compromised extension with broad access could then leak data to a third-party server. Imagine a seemingly innocuous calendar extension requesting access to your email, contacts, and financial information – a clear red flag indicating potential misuse. This excessive access provides ample opportunity for data exfiltration, allowing attackers to harvest personal details, financial data, or even sensitive communications.
Insecure Storage of OAuth Tokens
OAuth tokens are essentially digital keys granting access to protected resources. If these tokens are not securely stored within the extension, they become vulnerable to theft. Malicious code injected into the extension (perhaps through a compromised update mechanism) could easily steal these tokens and gain unauthorized access to the user’s accounts on the affected services. Consequences range from account takeover to data breaches, depending on the services connected using the compromised tokens. For example, a compromised extension storing tokens for a banking website could allow an attacker to access the user’s account and drain their funds.
Insufficient Input Validation and Sanitization
Many OAuth flows involve user interaction, such as entering usernames and passwords or approving access requests. If an extension fails to properly validate and sanitize user inputs during these interactions, it opens itself to various attacks. For example, a cross-site scripting (XSS) vulnerability could allow an attacker to inject malicious JavaScript code, potentially stealing OAuth tokens or manipulating the authentication flow to redirect the user to a phishing site. This could lead to the user unknowingly compromising their credentials, granting attackers full access to their accounts. Real-world examples exist where extensions have fallen prey to such vulnerabilities, resulting in large-scale data breaches and user account compromises. These breaches can have significant repercussions for both the users and the services they use.
Secure Coding Practices for OAuth in Chrome Extensions
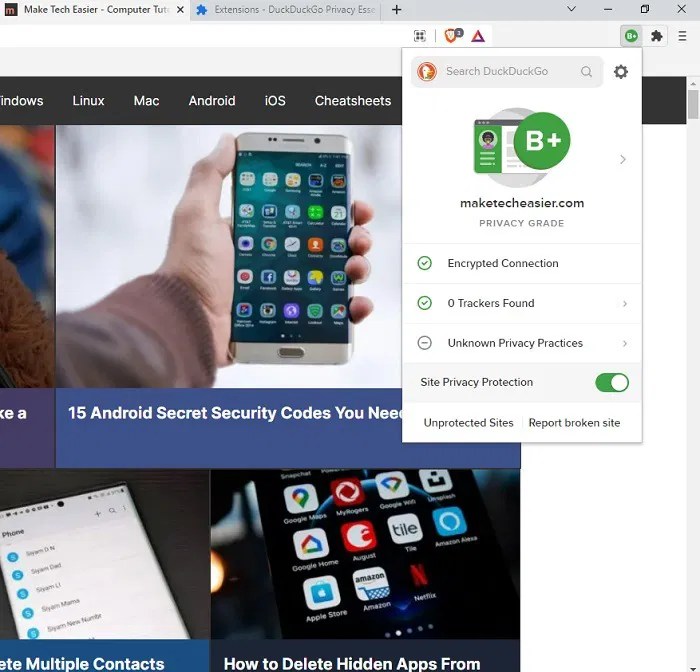
Source: maketecheasier.com
So, you’ve built a killer Chrome extension that needs access to user data through OAuth 2.0. Awesome! But before you unleash it on the world, let’s make sure it’s as secure as Fort Knox. Ignoring security best practices can lead to disastrous consequences, exposing sensitive user information and potentially damaging your reputation. This section dives into the crucial aspects of secure coding practices for OAuth within your Chrome extension.
OAuth security in extensions isn’t just about ticking boxes; it’s about building a robust, resilient system that protects user data at every stage. We’ll cover token validation, storage, permission management, and more, providing practical examples to help you build a truly secure extension.
OAuth Token Validation and Replay Attack Prevention
Validating OAuth tokens is paramount. A simple check isn’t enough; you need a multi-layered approach. First, verify the token’s signature to ensure it hasn’t been tampered with. Next, check the token’s expiration time to prevent expired tokens from granting access. Crucially, implement mechanisms to prevent token replay attacks. This involves keeping a record of used tokens (perhaps in a local database or using a server-side component) and rejecting any token that has already been used. A robust solution might involve incorporating a nonce (a random number used only once) within the token generation process to further enhance security. Failing to validate tokens properly leaves your extension vulnerable to unauthorized access.
Secure Storage and Handling of OAuth Tokens
How you store and handle OAuth tokens is critical. Never store tokens directly in plain text within your extension’s code or local storage. Instead, utilize the Chrome Storage API’s encrypted storage options. This adds a layer of protection, making it significantly harder for attackers to extract tokens even if they compromise your extension’s code. Consider using a robust encryption library and employing strong, randomly generated keys for enhanced security. Regularly review and update your encryption practices to keep up with evolving security standards. Remember, the goal is to make it as difficult as possible for malicious actors to gain access to sensitive user data.
Best Practices for Requesting and Managing User Permissions
When requesting permissions, be explicit and only request the absolute minimum necessary. Avoid requesting broad permissions if your extension only needs access to specific data points. Users are more likely to grant permission when they understand precisely what data your extension needs. Clearly explain the reasons for each permission request within your extension’s settings or consent flow. Regularly review and update your permission requests to ensure they align with your extension’s functionality. Overly broad or unnecessary permissions raise red flags and erode user trust. Transparency and a minimalist approach to permission requests are key to building a secure and trustworthy extension.
Analyzing the Manifest File for Potential OAuth Vulnerabilities
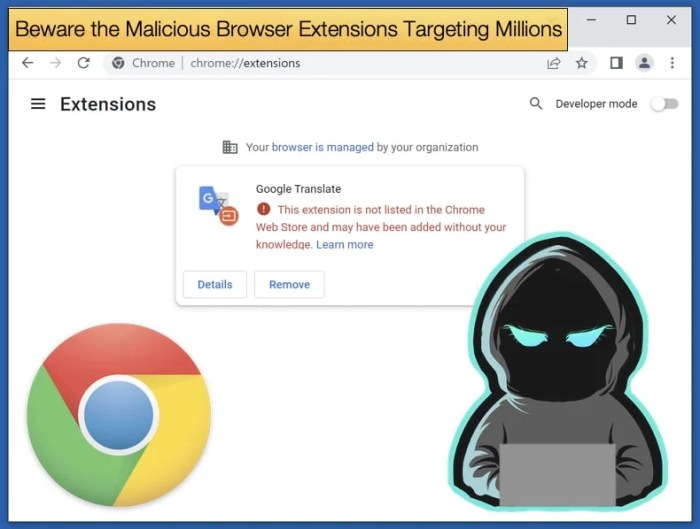
Source: or.id
The manifest file (manifest.json) is the heart of every Chrome extension, detailing its capabilities and permissions. A thorough examination of this file is crucial for identifying potential OAuth vulnerabilities before they can be exploited. Ignoring this step leaves your extension vulnerable to malicious attacks that could compromise user data. Think of it as a security blueprint – a poorly designed one can lead to a catastrophic breach.
The manifest file acts as a gatekeeper, defining what resources a Chrome extension can access. Understanding its structure and the permissions it requests is vital for assessing the security of an OAuth implementation. This involves carefully scrutinizing the permissions requested and comparing them against the actual functionality of the extension. Any discrepancy could signal a potential security risk.
The Significance of the `permissions` Field
The `permissions` field within the manifest.json file is a list specifying the capabilities your extension requires. It’s a crucial security element because it dictates the level of access the extension has to the user’s system and data. For OAuth integrations, this field is particularly important, as it defines whether the extension has permission to access sensitive user information or interact with specific websites. An overly broad `permissions` field could grant unnecessary privileges, potentially allowing attackers to leverage the extension for malicious purposes.
Suspicious Permissions Indicating Potential OAuth Vulnerabilities
Certain permissions in the `permissions` field should raise immediate red flags when analyzing a Chrome extension’s security posture, especially concerning OAuth. These permissions, if not strictly necessary for the extension’s intended functionality, might indicate malicious intent or poor security practices.
"activeTab"
: While seemingly benign, this permission, combined with others, could allow an extension to access sensitive information from a user’s currently active tab, potentially interfering with OAuth flows. It could be used to capture OAuth tokens or manipulate authentication processes."storage"
: Access to local or sync storage could be used to store OAuth tokens insecurely, making them vulnerable to theft. If the extension doesn’t explicitly need persistent storage for legitimate functionality, this permission is suspicious."identity"
or"identity.email"
: These permissions provide access to the user’s identity information, including their email address. While sometimes necessary, over-permissioning here is a major concern, especially when combined with other permissions like access to specific web APIs."tabs"
: Broad access to tabs can be abused to intercept OAuth redirects or manipulate the user’s browsing experience to steal credentials."*://*/*"
(Wildcard permissions): This grants access to all websites and resources, making it a massive security risk. It should almost never be used in a production extension.
Checklist for Reviewing the Manifest File for OAuth Security
A structured approach is essential when analyzing a manifest file for potential OAuth vulnerabilities. The following checklist helps ensure a comprehensive review.
- Verify Necessity: For each permission listed, determine if it’s absolutely necessary for the extension’s core functionality. Any unnecessary permissions should be immediately flagged.
- Minimize Scope: Avoid wildcard permissions (`”*://*/*”`) whenever possible. Specify the exact URLs or API endpoints the extension requires access to.
- OAuth Token Handling: Check for any indication of how OAuth tokens are handled. Ideally, tokens should be stored securely using Chrome’s storage APIs with appropriate encryption.
- Review Third-Party Libraries: If the extension utilizes third-party libraries, review their security practices and ensure they don’t introduce vulnerabilities.
- Test for Privilege Escalation: Attempt to identify scenarios where the extension could escalate its privileges beyond what’s explicitly granted in the manifest file.
Mitigation Strategies and Best Practices
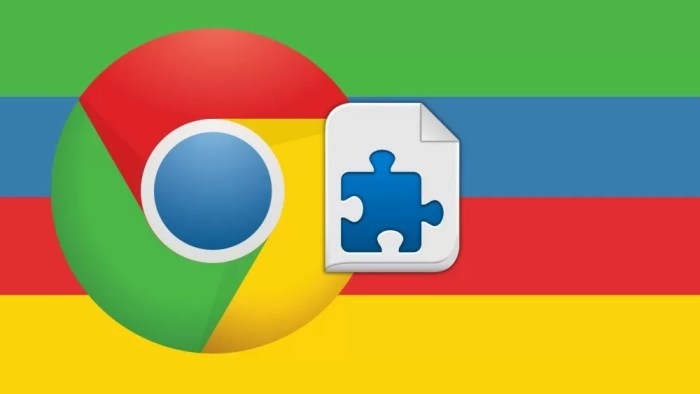
Source: techspot.com
OAuth vulnerabilities in Chrome extensions can be a serious security risk, potentially granting malicious actors access to sensitive user data. Fortunately, a multi-pronged approach encompassing robust coding practices, proactive security measures, and diligent maintenance can significantly reduce this risk. This section explores effective mitigation strategies and best practices to secure your extensions.
Robust Error Handling in OAuth Flows, Oauth vulnerability in chrome extensions
Proper error handling is crucial for preventing vulnerabilities and providing a better user experience. Instead of simply displaying generic error messages, developers should implement detailed error handling that provides informative feedback to both the user and the developer. This includes logging specific error codes and messages, and handling potential exceptions gracefully. For instance, if an authorization request fails, the extension should log the error details (e.g., HTTP status code, error message from the authorization server) and inform the user about the problem in a clear and concise manner, guiding them towards potential solutions (e.g., checking their internet connection, verifying their credentials). This detailed logging aids in debugging and identifying potential vulnerabilities. Furthermore, the extension should prevent sensitive information, such as refresh tokens, from being inadvertently exposed in error messages.
Regular Updates and Patching
Staying up-to-date with the latest security patches is paramount. Regularly updating your extension’s codebase and all third-party libraries used for OAuth implementation is essential. Outdated libraries often contain known vulnerabilities that can be exploited by attackers. A scheduled update process, perhaps tied to a version control system like Git, ensures consistent patching and minimizes the window of vulnerability. Furthermore, subscribing to security advisories from relevant sources, such as the Chromium project, allows developers to quickly address newly discovered vulnerabilities.
Tools and Techniques for Detecting and Preventing OAuth Attacks
Several tools and techniques can help developers detect and prevent OAuth attacks in their Chrome extensions. Static analysis tools can scan the extension’s code for potential vulnerabilities, including insecure OAuth implementations. Dynamic analysis tools can monitor the extension’s runtime behavior, identifying suspicious activities like unauthorized access attempts. Security testing frameworks, such as OWASP ZAP, can simulate attacks and assess the extension’s resilience. Additionally, employing code review practices and using linters that enforce secure coding standards can significantly reduce the likelihood of vulnerabilities. Finally, regularly auditing the extension’s permissions and ensuring they are strictly limited to what is necessary enhances security. For example, if an extension only needs to access user’s profile information, it shouldn’t request access to their entire Google Drive.
Illustrative Example of a Vulnerable Extension
Let’s imagine a fictional Chrome extension, “SuperSocialSharer,” designed to streamline sharing content across various social media platforms. This extension uses OAuth 2.0 to allow users to connect their accounts without directly sharing their passwords. However, a critical vulnerability lurks within its implementation.
The extension requests broad permissions during the OAuth flow, including access to all user data on connected social media platforms, not just the data necessary for sharing. This over-permissioning is a major security flaw.
Vulnerable OAuth Implementation Details
SuperSocialSharer’s OAuth implementation fails to properly validate the tokens it receives from the social media providers. A malicious actor could potentially craft a fake access token, mimicking a legitimate one. This token wouldn’t be detected as fraudulent by the extension’s inadequate verification mechanisms.
Exploitation by a Malicious Actor
A malicious actor, let’s call him “Mal,” crafts a fake Chrome extension, “SuperSocialSharer-Improved,” which closely resembles the legitimate extension. This malicious extension contains a backdoor that silently sends all obtained data to a server controlled by Mal. Crucially, “SuperSocialSharer-Improved” leverages the same OAuth flow, but instead of sending the user to the legitimate social media authentication pages, it redirects them to a fake login page mimicking the real ones. This fake page collects the user’s credentials, which are then used to obtain a legitimate access token.
Data Breach Sequence
1. User Installs: A user downloads and installs “SuperSocialSharer-Improved,” believing it to be the legitimate extension.
2. Fake OAuth Flow: The extension initiates the OAuth flow, redirecting the user to Mal’s fake login page.
3. Credential Capture: The user unknowingly enters their credentials on the fake page.
4. Token Acquisition: Mal uses the stolen credentials to obtain a legitimate access token from the social media provider.
5. Data Exfiltration: “SuperSocialSharer-Improved” uses this legitimate token to access the user’s social media data (posts, messages, contacts, etc.) and silently transmits it to Mal’s server.
6. Data Breach: The user’s private information is now in Mal’s possession.
Impact of the Vulnerability
The compromised data could include everything from personal messages and private photos to sensitive financial information linked to the user’s social media accounts. This information could be used for identity theft, blackmail, targeted advertising, or other malicious purposes. The scale of the breach depends on the number of users who installed the malicious extension and the level of access granted by the compromised accounts. The damage could range from minor privacy violations to significant financial and reputational harm.
Last Word
The seemingly innocuous Chrome extension can be a Trojan horse, silently jeopardizing your online security. Understanding the intricacies of OAuth vulnerabilities is crucial for navigating the digital landscape safely. By diligently analyzing manifest files, employing secure coding practices, and staying updated with the latest security patches, you can significantly mitigate the risks associated with OAuth vulnerabilities in Chrome extensions. Remember, vigilance is your best defense in the ever-evolving world of online threats. Don’t let a convenient extension become your worst digital nightmare.