Memory based attacks are the silent ninjas of the digital world, stealthily exploiting vulnerabilities in software to wreak havoc. These attacks target a system’s memory, manipulating data or code execution to gain unauthorized access or control. From subtle data breaches to full-blown system compromises, the impact can be devastating, highlighting the critical need for robust security measures. This deep dive explores the various techniques used, the defenses available, and real-world examples that showcase the ever-evolving nature of this threat landscape.
We’ll unpack the intricacies of different attack types, from classic buffer overflows to the more sophisticated return-oriented programming (ROP). We’ll delve into the technical details, providing clear explanations and code examples to illustrate vulnerable functions. But it’s not all doom and gloom; we’ll also explore effective defense mechanisms, including secure coding practices and memory protection techniques like ASLR and DEP. Finally, we’ll analyze real-world case studies to provide a practical understanding of how these attacks unfold and what lessons can be learned.
Introduction to Memory-Based Attacks
Memory-based attacks, a sneaky breed of cybercrime, exploit vulnerabilities in a system’s memory to gain unauthorized access or disrupt its operations. They’re like digital pickpockets, silently snatching sensitive information or wreaking havoc without leaving much of a trace. Unlike attacks targeting network vulnerabilities, these focus on the system’s internal workings, making them particularly insidious. Understanding how they operate is crucial for building robust security defenses.
Memory-based attacks leverage weaknesses in how a computer system manages and protects its memory – the temporary storage space where data and instructions reside while the system is running. By manipulating this memory, attackers can gain control of processes, steal sensitive data, or even crash the entire system. The consequences can range from minor inconveniences to catastrophic data breaches and complete system failures.
Types of Memory-Based Attacks
Several distinct types of memory-based attacks exist, each with its own unique methodology and target. These attacks often exploit vulnerabilities in programming languages, operating systems, or applications. The sophistication of these attacks varies greatly, from relatively simple exploits to highly complex, targeted attacks.
Examples of Real-World Memory-Based Attacks
The infamous Heartbleed bug, a vulnerability in OpenSSL, is a prime example of a memory-based attack. This bug allowed attackers to read memory containing sensitive information like private keys and passwords from vulnerable servers. The impact was widespread, affecting countless organizations and individuals. Another example is the use of buffer overflow attacks, where attackers flood a system’s buffer with excessive data, overflowing it and potentially overwriting critical memory locations, allowing them to inject malicious code. These attacks have been used for years to compromise systems and gain control.
Comparison of Memory-Based Attack Techniques
Attack Name | Target | Method | Mitigation |
---|---|---|---|
Buffer Overflow | Application memory | Overwriting memory buffers with excessive data to inject malicious code. | Secure coding practices, input validation, address space layout randomization (ASLR). |
Heap Spraying | Heap memory | Filling the heap memory with shellcode to increase the chance of execution. | Data Execution Prevention (DEP), ASLR, heap protection mechanisms. |
Return-oriented Programming (ROP) | Return addresses on the stack | Chaining together existing code snippets to execute arbitrary commands. | Control Flow Integrity (CFI), ASLR, improved compiler optimizations. |
Use-After-Free | Freed memory | Accessing memory that has already been freed, potentially leading to arbitrary code execution. | Careful memory management, garbage collection, and robust programming practices. |
Memory Exploitation Techniques
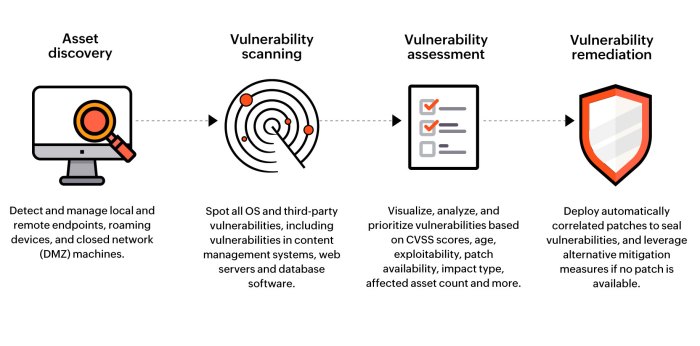
Source: manageengine.com
Memory exploitation techniques are the dirty tricks hackers use to manipulate a program’s memory, often leading to crashes, data breaches, or even complete system takeover. These attacks exploit vulnerabilities in how software handles memory allocation and access, turning seemingly innocuous coding errors into powerful weapons. Understanding these techniques is crucial for developers to write secure code and for security professionals to defend against these attacks.
Buffer Overflow Attacks
Buffer overflow attacks are a classic example of memory exploitation. They occur when a program attempts to write data beyond the allocated buffer size, overwriting adjacent memory regions. This can lead to unpredictable behavior, including program crashes, arbitrary code execution, and data corruption. Variations include stack-based buffer overflows (targeting the program’s stack) and heap-based buffer overflows (targeting the program’s heap). The severity depends on what is overwritten – if critical program data or return addresses are overwritten, the attacker gains control.
Heap-Based Overflow Attacks
Heap-based overflow attacks target the heap, a region of memory used for dynamic memory allocation. Unlike stack-based overflows, which are often easier to predict due to the stack’s predictable structure, heap-based overflows are more challenging to exploit due to the heap’s dynamic nature. The attacker needs to understand how the heap is managed and identify vulnerabilities in memory allocation and deallocation functions. A successful attack might involve corrupting metadata associated with allocated blocks, leading to memory corruption or allowing the attacker to allocate memory in unexpected locations, potentially leading to code execution.
Examples of Vulnerable Code
Here are some code examples demonstrating vulnerabilities susceptible to memory-based attacks. Note that these examples are simplified for illustrative purposes and should not be used in production code.
Vulnerable C function (stack overflow):
void vulnerable_function(char *input) int main()
#include
#include
char buffer[16];
strcpy(buffer, input); // Vulnerable: No bounds checking
printf("You entered: %s\n", buffer);
char long_input[32] = "This input is longer than the buffer!";
vulnerable_function(long_input);
return 0;
Vulnerable C++ function (heap overflow):
void vulnerable_function(int size) int main()
#include
#include
char *buffer = new char[size]; // Vulnerable: Size not checked
std::string long_string(size * 2, 'A'); // Create a string twice the size
strcpy(buffer, long_string.c_str()); // Copy into the smaller buffer
delete[] buffer;
vulnerable_function(10);
return 0;
These examples highlight the importance of input validation and bounds checking to prevent memory-based attacks. Failing to perform these checks can leave your application wide open to exploitation. Remember, security is not an afterthought – it’s a fundamental aspect of software development.
Defense Mechanisms Against Memory-Based Attacks
Memory-based attacks exploit vulnerabilities in how software handles memory, leading to crashes, data breaches, or even complete system compromise. Fortunately, numerous defense mechanisms exist to mitigate these threats, significantly improving software security. Understanding and implementing these defenses is crucial for building robust and resilient applications.
Several layers of protection can be employed to thwart memory-based attacks. These range from hardware-level features to software development practices and security tools. Effective defense often involves a multi-layered approach, combining various techniques to create a robust security posture.
Address Space Layout Randomization (ASLR)
ASLR randomizes the base addresses of key memory regions, such as the stack, heap, and libraries, making it difficult for attackers to predict the location of critical data structures. This significantly hinders return-oriented programming (ROP) attacks, a common exploitation technique that relies on chaining together existing code snippets. By making the target addresses unpredictable, ASLR increases the difficulty of launching successful attacks. For example, if an attacker’s exploit relies on jumping to a specific address within a library, ASLR makes this address change with each program execution, rendering the exploit ineffective.
Data Execution Prevention (DEP)
DEP, also known as the NX bit (No-eXecute bit), is a hardware-based protection mechanism that prevents code from being executed from memory regions designated as data. This directly counters attacks that attempt to inject malicious code into the data segment (e.g., the stack or heap) and then execute it. By marking these areas as non-executable, DEP stops the malicious code from running, thus neutralizing the attack. A common example of this is a buffer overflow attack where an attacker attempts to overwrite the return address on the stack with the address of their malicious code. DEP would prevent the execution of this code.
Control Flow Integrity (CFI)
CFI is a more advanced technique that verifies the integrity of a program’s control flow. It works by tracking the legitimate paths of execution within the program and preventing any unexpected jumps or calls. This effectively stops return-oriented programming (ROP) attacks, which rely on redirecting the program’s control flow to arbitrary locations. CFI enforces a strict set of rules on how the program can transition between different code sections, preventing malicious alterations to the control flow. For instance, if an attacker tries to hijack the control flow through a return-oriented programming attack, CFI will detect this deviation and terminate the program.
Secure Coding Strategies to Mitigate Memory-Based Attacks
Developing secure code is paramount in preventing memory-based vulnerabilities. This involves adhering to specific coding practices and utilizing secure programming libraries. Neglecting these practices dramatically increases the likelihood of vulnerabilities.
A secure coding strategy emphasizes preventing buffer overflows, using safe string handling functions, and performing thorough input validation. It also includes careful memory management to avoid dangling pointers and memory leaks.
Best Practices for Secure Software Development
Implementing a comprehensive set of best practices is essential for developing secure software. These practices aim to minimize vulnerabilities and enhance the overall security posture of the application.
- Input Validation: Always validate all user inputs to prevent injection attacks and ensure data integrity.
- Safe String Handling: Utilize safe string handling functions to prevent buffer overflows and other memory-related issues.
- Memory Management: Employ proper memory allocation and deallocation techniques to avoid memory leaks and dangling pointers.
- Secure Libraries: Use well-vetted and secure libraries, regularly updating them to patch known vulnerabilities.
- Regular Security Audits: Conduct periodic security audits and penetration testing to identify and address potential vulnerabilities.
- Code Reviews: Implement thorough code reviews to catch vulnerabilities before they reach production.
- Compiler Optimizations: Utilize compiler optimizations such as stack canaries to detect buffer overflows.
- Static and Dynamic Analysis Tools: Employ static and dynamic analysis tools to identify potential vulnerabilities in the codebase.
Advanced Memory-Based Attacks
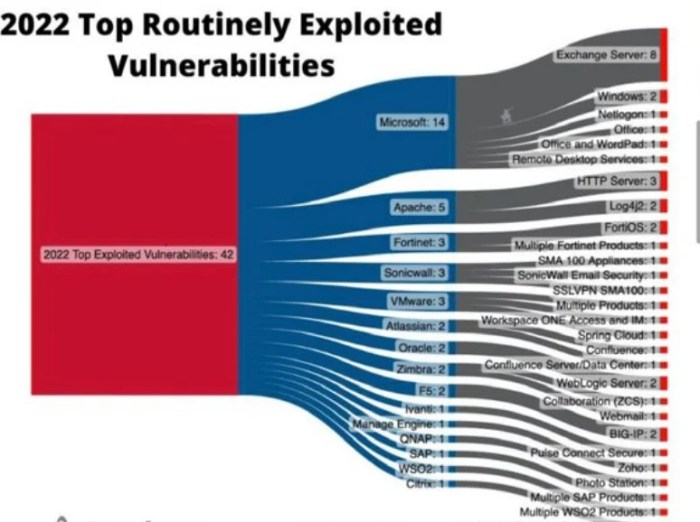
Source: antidos.com
Beyond the basics of buffer overflows and heap sprays lie more sophisticated memory-based attacks that exploit vulnerabilities at a deeper level. These advanced techniques often require a more intricate understanding of program execution and memory management, making them harder to detect and defend against. This section delves into one such technique: Return-Oriented Programming (ROP).
Return-Oriented Programming is a powerful attack technique that circumvents traditional defenses like address space layout randomization (ASLR) and data execution prevention (DEP). Instead of injecting malicious code directly into memory, ROP chains together short sequences of existing code – “gadgets” – to achieve arbitrary code execution. These gadgets typically end with a `ret` instruction, which transfers control to the next gadget in the chain.
Return-Oriented Programming (ROP)
ROP leverages existing code snippets within a program’s memory space. Attackers carefully select and chain together these “gadgets,” each ending with a `ret` instruction. This allows them to manipulate the program’s control flow without directly executing injected malicious code. The selection of gadgets is crucial; the attacker must find a sequence that performs the desired operations, such as opening a network connection, reading a file, or executing a shell command. The process is complex, often requiring sophisticated tools and a deep understanding of the target program’s binary code. A successful ROP attack can lead to complete compromise of the system.
ROP Techniques
The core of a ROP attack lies in identifying and chaining suitable gadgets. This involves analyzing the target program’s memory to locate code sequences that perform specific operations, followed by careful construction of the ROP chain. This chain is meticulously crafted to control the program’s execution flow, redirecting it to perform malicious actions. The attacker must accurately predict the addresses of the gadgets and carefully manage the stack to ensure proper execution of the chain. This process often involves exploiting vulnerabilities that allow for arbitrary writing to the stack, such as buffer overflows. Tools like ROPgadget help automate parts of this process by identifying potential gadgets within a binary.
Control-Flow Integrity (CFI) as a Defense Against ROP
Control-Flow Integrity (CFI) is a powerful defense mechanism designed to mitigate ROP and other control-flow hijacking attacks. CFI works by enforcing restrictions on the program’s control flow. It verifies that all control transfers, including function calls and returns, are legitimate and conform to a predefined control-flow graph. If a control transfer attempts to jump to an unexpected location, the CFI mechanism will detect and prevent it, effectively thwarting ROP attacks. Different CFI implementations exist, ranging from binary instrumentation to hardware-assisted solutions, each with its own trade-offs in performance and security. However, highly sophisticated attacks may still be able to bypass some CFI implementations.
Hypothetical Advanced Memory-Based Attack Scenario
Imagine a web server application vulnerable to a buffer overflow in its URL parsing function. An attacker crafts a specially crafted URL containing a malicious payload that exceeds the buffer size. This overflow overwrites the return address on the stack, redirecting execution to a carefully constructed ROP chain residing within the server’s memory. This chain consists of several gadgets: one to open a network socket, another to read data from a remote attacker-controlled server, and finally, one to execute the received data, which may be a shell command providing the attacker with complete control over the server. The impact could range from data theft and system compromise to a complete denial-of-service attack, depending on the attacker’s goals. The success of this attack relies on the attacker’s ability to find suitable gadgets within the server’s memory and to carefully craft the ROP chain to execute the desired sequence of actions. The lack of robust CFI mechanisms would significantly increase the likelihood of success for such an attack.
Forensic Analysis of Memory-Based Attacks: Memory Based Attacks
Memory forensics plays a crucial role in uncovering the digital breadcrumbs left behind by malicious actors exploiting memory vulnerabilities. Analyzing memory dumps allows investigators to reconstruct the attacker’s actions, identify the exploited vulnerabilities, and ultimately prevent future attacks. This process requires specialized tools and a methodical approach, as volatile memory data is ephemeral and easily altered.
The process involves acquiring a memory image, typically from a compromised system, and then meticulously examining its contents for evidence of malicious activity. This evidence might include injected code, manipulated data structures, or traces of unusual system calls. The analysis requires a deep understanding of operating system internals, memory management, and common attack techniques.
Memory Dump Acquisition and Analysis Techniques
Acquiring a memory dump is the first critical step. This can be done using various methods, ranging from live memory acquisition tools like Volatility or FTK Imager to post-mortem acquisition using tools provided by the operating system itself. The choice of method depends on the circumstances and the availability of access to the compromised system. Once acquired, the dump is then analyzed using specialized memory forensics tools. These tools provide functionalities to search for specific patterns, identify running processes, examine memory mappings, and analyze network connections. Advanced techniques might involve reconstructing the execution flow of malicious code or identifying the specific memory regions manipulated by the attack.
Tools Used in Memory Forensics
Several powerful tools facilitate the analysis of memory dumps. Volatility, a widely used open-source framework, provides a comprehensive suite of plugins for analyzing various aspects of memory, including processes, network connections, and loaded modules. Other tools like Rekall (a successor to Volatility), and The Sleuth Kit (TSK) are also commonly employed, often in conjunction with other digital forensics tools. The choice of tool often depends on the specific operating system and the type of attack being investigated.
Step-by-Step Procedure for Analyzing a Memory Image
A typical analysis workflow involves several sequential steps. First, the memory image is verified for integrity to ensure its authenticity and prevent tampering. Next, the investigator identifies running processes and examines their memory mappings for any suspicious code or data. Then, a deep dive into the process memory might reveal shellcode, injected libraries, or modified system calls. Network connections are also examined for evidence of communication with command-and-control servers. Finally, the timeline of events is reconstructed to understand the attack’s progression. This might involve correlating timestamps from various memory artifacts to build a comprehensive picture of the attack.
Common Artifacts Found in Memory During a Memory-Based Attack Investigation
Artifact Type | Location | Significance | Detection Method |
---|---|---|---|
Shellcode | Process memory space | Indicates malicious code execution | String/pattern matching, code analysis |
Hooked API calls | Import Address Table (IAT) | Suggests manipulation of system functions | IAT analysis, memory scanning |
Modified system variables | Kernel memory space | May indicate privilege escalation | Memory scanning, kernel analysis |
Network connections | Network stack | Reveals communication with external servers | Network connection analysis |
Case Studies of Memory-Based Attacks
Memory-based attacks, exploiting vulnerabilities in how a system manages its memory, have proven devastatingly effective in the real world. Understanding these attacks through real-world examples allows us to better appreciate the sophistication of these techniques and the critical need for robust defenses. The following case studies highlight diverse approaches, exploited vulnerabilities, and the resulting impact.
The Heartbleed Bug
The Heartbleed vulnerability (CVE-2014-0160), discovered in OpenSSL in 2014, allowed attackers to extract sensitive data from the memory of affected servers. The bug resided in the heartbeat extension of the OpenSSL library, a feature intended for efficient communication. Attackers could send a specially crafted heartbeat request that requested more data than was actually sent, causing the server to return a chunk of its memory. This chunk could contain private keys, passwords, user data, and other sensitive information. The impact was widespread, affecting countless websites and services globally, highlighting the catastrophic consequences of a single, seemingly minor vulnerability. The vulnerability exploited a lack of bounds checking within the heartbeat extension’s code.
The Shellshock Vulnerability
The Shellshock vulnerability (CVE-2014-6271 and CVE-2014-7169), affecting the Bash shell, demonstrated the potential for remote code execution via memory manipulation. A specific environment variable, improperly handled by Bash, allowed attackers to inject arbitrary code into the shell’s memory space. This allowed for remote code execution, granting attackers complete control over the affected system. The vulnerability’s impact was significant, affecting numerous systems running Bash, from servers to embedded devices. The root cause lay in the insecure parsing of environment variables by the Bash shell interpreter, leading to unintended code execution within the program’s memory.
The Equation Group Attacks
The Equation Group, a sophisticated advanced persistent threat (APT) actor, utilized various memory-based attack techniques, including sophisticated exploits leveraging vulnerabilities in hardware and software to gain access to and exfiltrate sensitive data. Their attacks involved exploiting vulnerabilities in the firmware of hard drives and other components, allowing them to gain persistent access and monitor systems even after a system reboot. These attacks highlight the potential for memory-based attacks to bypass traditional security measures and establish long-term persistence within a target system. The precise vulnerabilities exploited by the Equation Group remain largely undisclosed, but their success underscores the increasing sophistication of these attacks and the need for comprehensive security measures that extend beyond software vulnerabilities.
Return-oriented Programming (ROP) Attacks, Memory based attacks
Return-oriented programming (ROP) is a sophisticated technique used to bypass traditional exploitation prevention methods such as address space layout randomization (ASLR). Instead of injecting malicious code directly into memory, ROP chains together existing code snippets (gadgets) within the program’s memory to execute arbitrary instructions. This allows attackers to achieve code execution even in environments where direct code injection is difficult or impossible. The effectiveness of ROP attacks underscores the need for more advanced defense mechanisms that go beyond simple memory protection techniques. Numerous real-world examples demonstrate the successful use of ROP in bypassing security measures and achieving code execution on vulnerable systems. The effectiveness stems from the reuse of existing code, making detection and prevention challenging.
Wrap-Up
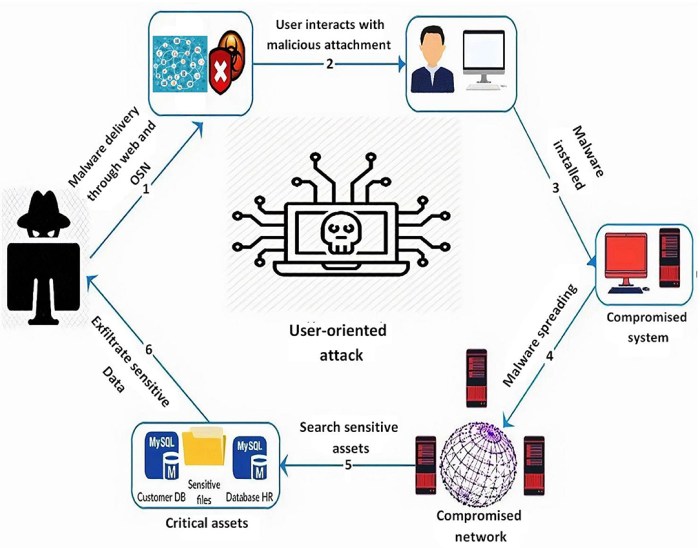
Source: frontiersin.org
Memory based attacks remain a significant threat, constantly evolving to bypass security measures. Understanding the underlying techniques, implementing robust defenses, and staying updated on the latest attack vectors are crucial for safeguarding systems. While the battle against these attacks is ongoing, a multi-layered approach combining secure coding practices, memory protection mechanisms, and diligent forensic analysis offers the best chance of staying ahead of the curve. The stakes are high, but with knowledge and vigilance, we can significantly reduce our vulnerability.