Angular expressions vulnerability: It sounds technical, right? But this sneaky security hole can expose your web app to nasty Cross-Site Scripting (XSS) attacks. Think of it like a backdoor – malicious code slips in, wreaking havoc on your users and your reputation. We’re diving deep into how these expressions work, how they can be exploited, and, most importantly, how to bulletproof your Angular apps against this threat.
This guide breaks down the intricacies of Angular expressions, exploring their functionality and the various ways they can be misused. We’ll cover common XSS vulnerabilities, effective sanitization techniques, and best practices for secure data binding. From understanding the different types of Angular expressions to implementing advanced mitigation strategies like Content Security Policy (CSP), we’ll equip you with the knowledge to build secure and robust Angular applications. We’ll even walk through real-world scenarios to illustrate both successful attacks and effective defenses.
Introduction to Angular Expressions
Angular expressions are the backbone of data binding in AngularJS applications. They’re snippets of JavaScript code that are embedded within Angular templates and evaluated by the AngularJS framework to dynamically update the view based on the underlying model. Think of them as the glue that connects your data to what the user sees on the screen. Without them, AngularJS would be a static, unchangeable webpage.
Angular expressions are primarily used to display data, perform calculations, and manipulate data within the template. They offer a concise and efficient way to manage the flow of information between your application’s model and the user interface, leading to dynamic and interactive web applications. Crucially, they differ from JavaScript expressions in several key aspects, primarily in their context and how they’re evaluated.
Angular Expression Types and Usage
Angular expressions support a variety of operations, mirroring many standard JavaScript functionalities. However, they’re designed specifically for the AngularJS context and have limitations compared to full JavaScript. They primarily handle data binding, allowing you to effortlessly display model data within the template. This eliminates the need for manual DOM manipulation, simplifying development and improving performance. For instance, you can directly display a model’s property value using double curly braces ` myModel.propertyName `. Beyond simple display, they can perform arithmetic operations, comparisons, and logical operations, enriching the dynamic capabilities of your templates.
Common Angular Expression Syntax
Angular expressions utilize a straightforward syntax that leverages standard JavaScript operators within the context of double curly braces ` `. This allows for seamless integration of data binding and dynamic content within your HTML. Here are a few examples illustrating common usage:
` 1 + 2 ` This expression evaluates to 3 and displays the result in the template.
` myModel.name ` This displays the value of the `name` property from the `myModel` object.
` myModel.age > 18 ? ‘Adult’ : ‘Minor’ ` This uses a ternary operator to display ‘Adult’ if `myModel.age` is greater than 18, otherwise ‘Minor’.
` myModel.products | filter: ‘shoes’ ` This shows the use of filters to modify data displayed. In this case, it filters `myModel.products` array to show only items containing “shoes”.
XSS Vulnerabilities in Angular Expressions
Angular expressions, while powerful for dynamic data binding, can become a sneaky backdoor for attackers if not handled carefully. They offer a direct pathway to inject malicious scripts into your application, leading to Cross-Site Scripting (XSS) vulnerabilities. Understanding how these vulnerabilities arise and implementing robust preventative measures is crucial for building secure Angular applications.
Improperly sanitized Angular expressions are a major source of XSS vulnerabilities. When user-supplied data is directly embedded into an expression without proper validation and escaping, an attacker can inject malicious JavaScript code that will execute within the context of the victim’s browser. This can lead to a range of harmful consequences, from stealing sensitive information to hijacking user sessions. The severity depends on the context of the injected script and the level of access the attacker gains.
Angular Expression Exploitation Methods
Attackers can exploit Angular expressions through various means, primarily by injecting malicious JavaScript code into data bound to the template. This often happens when user input is directly incorporated into the expression without adequate sanitization. For instance, if a user’s name is displayed using `userName` and the user inputs `` as their name, this script will execute in the browser. The severity of the attack is directly tied to the context of the data and the attacker’s capabilities. They could steal cookies, redirect users to phishing sites, or even modify the application’s behavior to their advantage.
Impact of Unsanitized Angular Expressions
The consequences of XSS vulnerabilities stemming from improperly sanitized Angular expressions can be severe. Successful exploitation can lead to data breaches, session hijacking, and website defacement. Attackers can gain unauthorized access to sensitive user data, such as passwords, credit card details, or personal information. They can also manipulate the application’s functionality to perform actions on behalf of the victim, like transferring funds or making unauthorized purchases. The impact is amplified when the application deals with sensitive data or performs critical actions on behalf of the user.
Examples of Vulnerable Angular Expressions and Exploits
Let’s illustrate with a simple example. Consider an Angular application displaying a user’s comment: `userComment`. If a user inputs ``, the `onerror` event will trigger an alert box, demonstrating a successful XSS attack. This is a basic example; more sophisticated attacks can involve stealing cookies, redirecting users, or even executing more complex malicious code. Another example could involve a search field: `searchQuery`. If an attacker inserts a malicious script, it could be executed within the context of the application, potentially leading to serious security breaches. These examples highlight the need for robust input sanitization.
Secure Coding Practices to Prevent XSS Vulnerabilities
Preventing XSS vulnerabilities requires a multi-layered approach. First and foremost, always sanitize user input before using it in Angular expressions. Angular provides built-in mechanisms like the `DomSanitizer` service to achieve this. This service allows you to safely render HTML, URLs, and scripts, preventing malicious code from being executed. Always use parameterized queries instead of directly embedding user input into SQL queries to avoid SQL injection vulnerabilities, which can be a vector for XSS attacks. Regular security audits and penetration testing are also crucial for identifying and addressing potential vulnerabilities. Furthermore, adopting a secure development lifecycle (SDLC) that incorporates security considerations at every stage of the development process is vital. Staying updated on the latest security best practices and using appropriate security tools can further enhance the security posture of your application.
Contextual Escaping and Sanitization in Angular
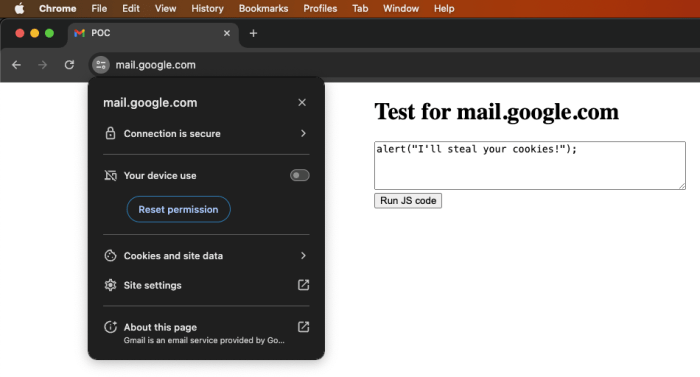
Source: medium.com
Angular, while a powerful framework, is susceptible to Cross-Site Scripting (XSS) vulnerabilities if not handled carefully. Improperly handling user-supplied data within Angular expressions can lead to malicious scripts executing in the browser, compromising user data and application security. Contextual escaping and sanitization are crucial defense mechanisms against these attacks. They act as gatekeepers, carefully inspecting and modifying potentially harmful input before it’s displayed on the page.
Contextual escaping and sanitization work by transforming potentially dangerous characters in user input into their harmless HTML entity equivalents. This prevents the browser from interpreting them as executable code. Angular provides a range of built-in tools and techniques to ensure data is rendered safely, based on its intended context (e.g., HTML, script, style, URL). The right sanitization method depends heavily on where the data is going to be displayed within your application.
Angular Sanitization Methods
Angular offers several sanitization methods, each tailored to a specific context. The primary method involves using built-in pipes like `DomSanitizer`. These pipes effectively transform potentially harmful input into a safe representation for display within specific HTML contexts. Incorrect use, however, can still lead to vulnerabilities, so understanding their limitations and capabilities is vital. For example, `bypassSecurityTrustHtml` should be used *extremely* cautiously, only when you are absolutely certain the data is safe. Misuse can easily negate all other security measures.
Comparing Angular Sanitization Pipes
Different sanitization pipes offer varying levels of protection. Choosing the right one is key to effective security. Using the wrong pipe might leave your application vulnerable. For instance, sanitizing a URL with an HTML sanitizer won’t prevent XSS if the URL itself contains malicious JavaScript.
Pipe | Context | Description | Example |
---|---|---|---|
DomSanitizer.bypassSecurityTrustHtml |
HTML | Bypasses security checks; use with extreme caution! Only use if you absolutely trust the source of the data. | domSanitizer.bypassSecurityTrustHtml(untrustedHtml) |
DomSanitizer.bypassSecurityTrustScript |
JavaScript | Bypasses security checks for JavaScript; extremely risky, generally avoid. | domSanitizer.bypassSecurityTrustScript(untrustedScript) |
DomSanitizer.bypassSecurityTrustStyle |
CSS | Bypasses security checks for CSS; use cautiously, only when the source is trusted. | domSanitizer.bypassSecurityTrustStyle(untrustedStyle) |
DomSanitizer.bypassSecurityTrustUrl |
URLs | Bypasses security checks for URLs; use with caution, ensure URL validation. | domSanitizer.bypassSecurityTrustUrl(untrustedUrl) |
Example: Securing User Input
Let’s say you have a user input field where users can enter text that will be displayed on the page. Without sanitization, this input could easily contain malicious JavaScript. Using the `DomSanitizer` pipe, however, we can effectively mitigate this risk. Consider this example:
“`typescript
import Component from ‘@angular/core’;
import DomSanitizer, SafeHtml from ‘@angular/platform-browser’;
@Component(
selector: ‘app-my-component’,
template: `
`
)
export class MyComponent
userInput: string = ”;
sanitizedInput: SafeHtml;
constructor(private domSanitizer: DomSanitizer)
ngOnInit()
this.sanitizedInput = this.domSanitizer.bypassSecurityTrustHtml(this.userInput); //Use cautiously!
“`
This example demonstrates the use of `bypassSecurityTrustHtml`. However, remember: this should only be used as a last resort and only when you have complete control and trust over the source of the `userInput` data. Ideally, you would perform thorough input validation and escaping before even considering this method.
Data Binding and Vulnerability Mitigation
Angular’s data binding mechanisms are powerful but require careful handling to avoid XSS vulnerabilities. Understanding how different binding types interact with user input and how to sanitize data is crucial for building secure applications. Improperly handled data binding can expose your application to attacks, leading to compromised user data and application functionality.
Angular offers several data binding techniques, each presenting a different level of risk regarding XSS. Interpolation, property binding, and event binding all handle data differently, and consequently, require different security considerations. Failing to adequately sanitize user-supplied data within these bindings can lead to the injection of malicious scripts.
Interpolation Binding and Security
Interpolation, using double curly braces ` expression `, directly inserts the value of an expression into the HTML template. This is the most straightforward binding type but also the most vulnerable to XSS attacks if the expression contains unsanitized user input. For example, if a user-provided username is directly interpolated without escaping, a malicious user could inject `` into their username, leading to a JavaScript alert box popping up on other users’ screens. This is because the browser interprets the injected script as executable code. Therefore, always sanitize data before interpolating it.
Property Binding and Security Considerations
Property binding, using `[property]=”expression”`, assigns the value of an expression to a property of a DOM element. While seemingly safer than interpolation, it still poses XSS risks if the bound property affects the HTML content. For instance, binding to the `innerHTML` property is extremely dangerous because it directly manipulates the HTML content, bypassing any built-in sanitization mechanisms. Instead, prefer binding to properties that don’t directly modify the HTML structure, or ensure thorough sanitization before binding to potentially risky properties.
Event Binding and XSS Mitigation
Event binding, using `(event)=”expression”`, allows you to execute code in response to DOM events. While not directly inserting data into the HTML, it’s important to sanitize any data received from event handlers before using it in other contexts. For example, if an event handler receives user input, that input should be sanitized before being displayed or used in other data bindings to prevent XSS. This ensures that even if malicious code is passed through an event, it won’t execute dangerously within the application.
Best Practices for Secure Data Binding in Angular
Safe data handling is paramount in Angular development. Failing to do so can have serious security implications. Following best practices ensures your application remains protected.
The following list Artikels crucial steps for mitigating XSS vulnerabilities related to data binding:
- Always sanitize user input: Before using any user-provided data in your templates, use Angular’s built-in sanitization pipes (e.g., `SafeHtmlPipe`, `SafeUrlPipe`, `SafeScriptPipe`) or the `DomSanitizer` service to sanitize the data according to its intended use. Never trust user input.
- Avoid `innerHTML` binding: Directly manipulating `innerHTML` is extremely risky and should be avoided whenever possible. Use Angular’s templating engine to create and manipulate the DOM safely.
- Use appropriate data binding types: Choose the binding type that best suits the situation. For static content, interpolation might be sufficient, but for dynamic updates, property or event binding may be necessary. Consider the security implications of each choice.
- Employ Content Security Policy (CSP): A robust CSP helps prevent XSS attacks even if some vulnerabilities exist in your application. It acts as a final layer of defense by restricting the resources the browser is allowed to load.
- Regular security audits and penetration testing: Regularly audit your code for potential vulnerabilities and conduct penetration testing to identify and fix security weaknesses before they can be exploited.
Secure Angular Expression Practices
Building secure Angular applications requires a proactive approach to handling user inputs. Ignoring security best practices can leave your application vulnerable to Cross-Site Scripting (XSS) attacks, potentially compromising user data and application integrity. This section details crucial steps to ensure your Angular expressions are safe and robust.
The core principle is to never trust user-supplied data. Always validate and sanitize any input before displaying it within your Angular templates. This prevents malicious code from being injected and executed within the browser.
Input Validation and Sanitization
Implementing robust input validation and sanitization is paramount. Validation ensures the data conforms to expected formats and constraints, while sanitization removes or neutralizes potentially harmful characters. This two-pronged approach significantly reduces the risk of XSS attacks.
Consider a scenario where a user can input their name into a form. A simple validation might check for a maximum length, ensuring the name doesn’t exceed a reasonable character limit. Sanitization, however, would go further, escaping special characters like `<`, `>`, and `&` to prevent them from being interpreted as HTML tags.
A step-by-step guide would involve:
- Define Validation Rules: Establish clear rules for acceptable input formats using regular expressions or custom validation functions. For example, a username might need to contain only alphanumeric characters.
- Implement Validation: Use Angular’s built-in validation features or create custom validators to enforce these rules. This can be done within your Angular components using the `Validators` module or custom validator functions.
- Sanitize Input: Employ Angular’s built-in sanitization pipes (like `DomSanitizer`) to safely transform potentially harmful input into safe HTML. This prevents the browser from interpreting malicious code as HTML.
- Display Sanitized Data: Only display data that has undergone both validation and sanitization within your templates. This final step ensures that even if a validation step is bypassed, the sanitization step provides an additional layer of protection.
Secure and Insecure Angular Expression Examples
Let’s illustrate the difference between secure and insecure practices.
Insecure Example:
userInput
Secure Example:
userInput | sanitizeHtml
In the secure example, the `sanitizeHtml` pipe (a placeholder for Angular’s actual sanitization mechanisms) would transform any potentially harmful HTML entities into their escaped equivalents, preventing code injection. Remember to use the appropriate Angular sanitization methods provided by the `DomSanitizer` service for different content types (HTML, script, style, URL, etc.).
Regular Security Audits and Penetration Testing
Proactive security measures are vital. Regular security audits and penetration testing identify vulnerabilities before attackers can exploit them. These assessments should be performed at various stages of development, including during design, implementation, and after deployment. The frequency depends on the application’s criticality and the potential impact of a breach. A well-defined security policy and regular training for developers are also crucial components of a robust security posture.
Advanced Mitigation Techniques
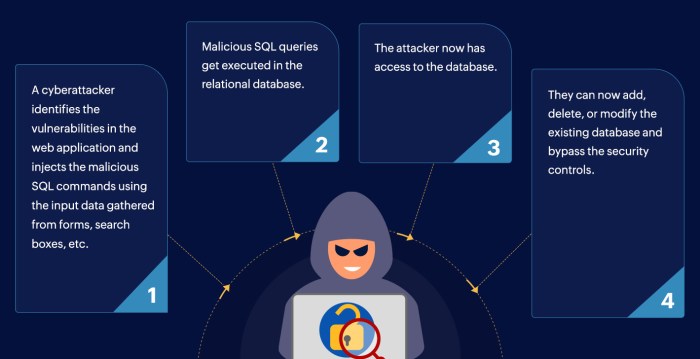
Source: manageengine.com
Beyond basic sanitization, bolstering your Angular application’s security requires a multi-layered approach. Implementing advanced techniques like Content Security Policy (CSP) significantly reduces the attack surface and minimizes the impact of successful XSS exploits. This involves proactively controlling the resources your application loads, limiting the potential for malicious code injection.
Content Security Policy (CSP) is a powerful mechanism that allows you to define a whitelist of sources from which your application is allowed to load resources, including scripts, stylesheets, images, and other assets. By restricting the sources, you effectively prevent the browser from loading resources from untrusted domains, thereby significantly mitigating the risk of XSS attacks. It acts as a final line of defense, even if other sanitization methods fail.
Content Security Policy Implementation
Implementing CSP involves adding a special HTTP response header, `Content-Security-Policy`, to your web server’s configuration. This header contains a set of directives that specify the allowed sources for different types of resources. For example, a directive like `script-src ‘self’` will only allow scripts from the same origin as the web application to be executed. Misconfiguration can inadvertently lock down functionality, so careful planning and testing are essential.
Example CSP Directives
The following examples illustrate how to configure CSP directives to protect against various XSS vulnerabilities:
To allow scripts only from your origin:
Content-Security-Policy: script-src 'self';
To allow scripts from a specific CDN:
Content-Security-Policy: script-src 'self' https://example-cdn.com;
To allow inline scripts (use with caution):
Content-Security-Policy: script-src 'self' 'unsafe-inline';
(Note: ‘unsafe-inline’ should be avoided whenever possible due to security risks.)
To allow images from anywhere:
Content-Security-Policy: img-src *;
(Note: Using ‘*’ is generally discouraged, prefer specific sources whenever feasible.)
A more comprehensive policy might look like this:
Content-Security-Policy: default-src 'self'; script-src 'self' 'unsafe-inline'; style-src 'self' 'unsafe-inline'; img-src 'self' data:; font-src 'self'; connect-src 'self'; object-src 'none'; base-uri 'self'; form-action 'self'; frame-ancestors 'none';
(Note: This is a sample; adapt it to your specific application needs. ‘unsafe-inline’ is used here for illustrative purposes only and should be avoided if possible.)
Reporting CSP Violations, Angular expressions vulnerability
To aid in identifying and fixing potential CSP violations, you can configure a report-uri directive. This sends reports of violations to a specified endpoint, allowing you to monitor and address any issues proactively. This proactive approach is crucial for continuous security improvement.
Content-Security-Policy: default-src 'self'; report-uri /csp-violation-report;
This example sends violation reports to the `/csp-violation-report` endpoint on your server. You’ll need to implement a handler to receive and process these reports.
Real-World Examples of Exploits: Angular Expressions Vulnerability
Let’s ditch the theory and dive into some real-world scenarios to see how Angular expression vulnerabilities can play out. Understanding these examples will help you build more secure Angular applications. We’ll look at a successful attack and a successful defense, highlighting the crucial differences.
AngularJS, while powerful, has a history of security concerns, primarily stemming from its flexible data binding mechanisms. Improperly sanitized user inputs, especially when directly embedded into the DOM using expressions, open the door for malicious actors.
Successful XSS Attack Through a Vulnerable Angular Expression
Imagine an e-commerce site built with AngularJS. A user profile page displays the username, fetched from a backend database, within an Angular expression like this: user.name
. This seems innocuous enough, but if the backend doesn’t properly sanitize the username before sending it to the frontend, an attacker could inject malicious JavaScript.
Let’s say an attacker manages to register with the username
. This seemingly harmless username contains an image tag with an onerror
event handler. When the user profile page renders, the browser attempts to load the image at URL “x”. Since this image likely doesn’t exist, the onerror
event fires, executing the JavaScript code and displaying the alert box. This simple example demonstrates how easily an attacker can inject malicious code and compromise the user’s session, steal cookies, or redirect the user to a phishing site.
The attack vector was the user registration process, the exploited vulnerability was the lack of input sanitization for usernames within the Angular expression, and the impact was the execution of arbitrary JavaScript code within the user’s browser context.
Successful Mitigation of an XSS Attempt
Now, let’s consider a different scenario. Another e-commerce site, this time built with careful consideration for security, uses the same user.name
expression to display usernames. However, they’ve implemented robust input sanitization on the backend. Before storing the username in the database, the application uses a function to escape any potentially harmful characters, effectively rendering them harmless.
Even if an attacker attempts the same attack as before, the sanitized username, perhaps something like <img src="x" onerror="alert('XSS Vulnerability!')">
, will be displayed literally as text, rendering the injected JavaScript code inert. The browser will correctly interpret the escaped characters as HTML entities, preventing the execution of any malicious code. This highlights the importance of server-side sanitization, as client-side sanitization alone is not always sufficient.
The security measures implemented included server-side input sanitization before data storage and rendering within the Angular expression. The effectiveness was complete prevention of the XSS attack, demonstrating that proactive security measures are paramount.
Ultimate Conclusion
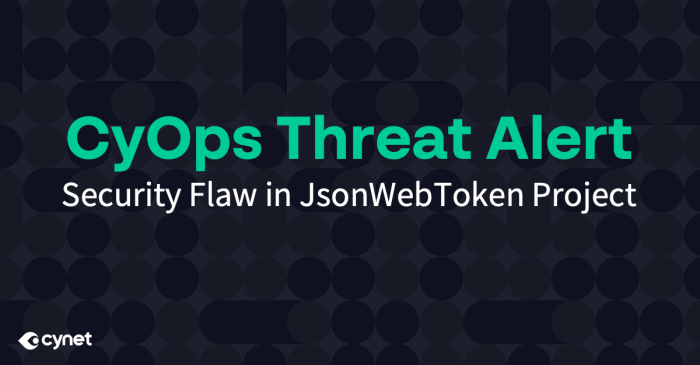
Source: cynet.com
Securing your Angular application against expression-based vulnerabilities is crucial. While the potential for XSS attacks is real, understanding the mechanics of Angular expressions, implementing robust sanitization methods, and adopting secure coding practices can significantly reduce your risk. Remember, proactive security measures – including regular security audits and penetration testing – are your best defense against this ever-evolving threat landscape. Don’t let a simple oversight leave your users vulnerable – take control of your app’s security today.